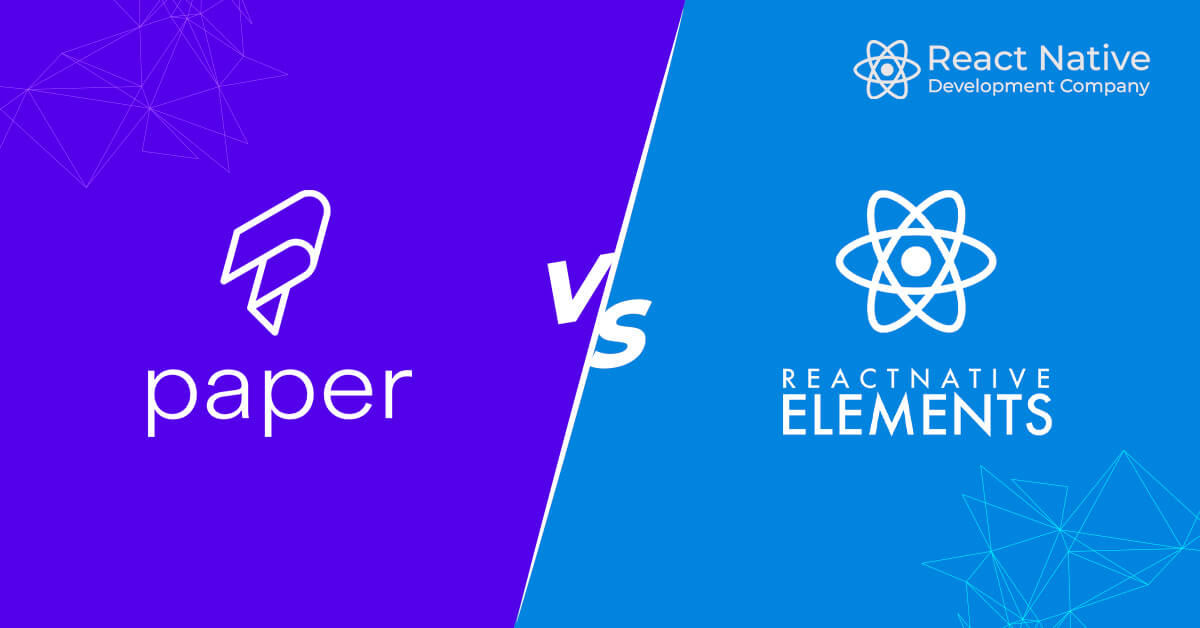
What is the difference between react-native paper and react-native elements?
Having a solid and effective foundation is essential in the rapidly changing world of mobile application development. React-Native Paper comes into play here. It is a widely acclaimed open-source UI framework specially crafted for React Native applications. With an extensive set of pre-built components, React-Native Paper enables developers to create visually appealing and intuitive user interfaces. This article aims to provide an in-depth exploration of React-Native-Paper, shedding light on its features, components, and the benefits it brings to the table.
Understanding React Native Paper
At its core, React Native Paper is a comprehensive UI framework that seamlessly integrates with React Native applications. It is designed to simplify the process of building high-quality user interfaces. Whether you are developing a social media platform, an e‑commerce website, or a productivity tool, React Native Paper proves to be a versatile and indispensable tool in your toolkit.
Key Features of React Native Paper
- Customizable Themes: One of the standout features of React Native Paper is its powerful theming system. This allows developers to effortlessly customize the visual aesthetics of their applications, ensuring a unique and engaging user experience.
- Icon Library: React Native Paper comes equipped with an extensive library of high-quality icons. This eliminates the need for external icon resources, streamlining the development process.
- Animated Elements: Adding a dynamic touch to your applications is made easy with React-Native-Paper. It provides seamless support for animations, enhancing the overall user experience.
- Accessibility Considerations: Accessibility is a critical aspect of any application. React-Native Paper is designed with accessibility in mind, ensuring that your app is usable by individuals with disabilities.
- Diverse Range of Components: From buttons and modals to data tables and icons, Paper React Native offers a rich collection of components. This extensive library accelerates the development process and maintains a consistent visual style.
Getting Started with React Native Paper
Implementing React Native Paper in your project is a straightforward process. Let’s walk through the essential steps:
Step 1: Installation
Begin by installing the react-native-paper package through npm or yarn.
[code]
npm install react-native-paper
[/code]
Step 2: Setting up the Theme
Configuring the theme for your application is crucial to maintaining a consistent visual style. Below is an example of how you can set up a basic theme:
[code]
import { Provider as PaperProvider } from ‘react-native-paper’;
const theme = {
…DefaultTheme,
roundness: 2,
colors: {
…DefaultTheme.colors,
primary: ‘#3498db’,
accent: ‘#f1c40f’,
},
};
export default function Main() {
return (
<PaperProvider theme={theme}>
{/* Your App Components */}
</PaperProvider>
);
}
[/code]
Step 3: Using Components
With React Native Paper integrated, you can start incorporating its components into your application. Here’s an example of how you can use a button component:
[code]
import { Button } from ‘react-native-paper’;
<Button mode=“contained” onPress={() => console.log(‘Pressed’)}>
Press me
</Button>
[/code]
Leveraging React Native Paper Icons
Icons are fundamental to enhancing the user experience. React Native Paper provides an extensive collection of icons that can be seamlessly integrated into your application. Here’s an example of how to incorporate an icon:
[code]
import { IconButton } from ‘react-native-paper’;
<IconButton icon=“star” color=“#FFD700” size={30} onPress={() => console.log(‘Star pressed’)} />
[/code]
Animations with React Native Paper
Adding animations to your application can greatly enhance its visual appeal. React Native Paper simplifies this process. Below is an example of how to add a Floating Action Button (FAB) with an animation:
[code]
import { View } from ‘react-native’;
import { FAB } from ‘react-native-paper’;
export default function MyComponent() {
return (
<View style={{ flex: 1, justifyContent: ‘center’, alignItems: ‘center’ }}>
<FAB
style={styles.fab}
icon=“plus”
onPress={() => console.log(‘FAB pressed’)}
/>
</View>
);
}
[/code]
React Native Paper is a powerful tool for building cross-platform mobile applications with a polished and consistent user interface. By leveraging its extensive range of components and features, developers can create stunning applications that provide an exceptional user experience.
What are React Native Elements?
React Native Elements is an open-source UI toolkit specifically designed for building mobile applications using the React Native framework. React Native itself is a popular JavaScript framework that enables developers to create cross-platform mobile apps with a single codebase, saving time and effort. React-Native-Elements takes this a step further by offering a set of pre-designed and highly customizable UI components, making it easier to create consistent and visually appealing interfaces for both iOS and Android platforms.
Features of React Native Elements:
- Cross-Platform Compatibility: React Native Elements is built to work seamlessly on both iOS and Android devices. This cross-platform compatibility ensures that your app looks and functions consistently across various devices, eliminating the need for separate development for each platform.
- Pre-Designed Components: The toolkit provides a comprehensive collection of pre-designed UI components, including buttons, input fields, headers, icons, and more. These components are ready to use “out of the box,” saving developers valuable time and effort in creating common app elements.
- Customization: While the components are pre-designed, they are also highly customizable. Developers can easily tailor these components to match the unique design requirements of their application. This flexibility allows for creative freedom without sacrificing development speed.
- Icon Support: React Native Elements includes a rich library of customizable icons that can be effortlessly integrated into your app’s user interface. These icons enhance the visual appeal of your app and improve user engagement.
- Consistency in Design: Maintaining a consistent design language across your app is crucial for providing a polished user experience. React-Native Elements enforce design consistency, ensuring that your app looks cohesive and professional.
- Active Community: Being open source, React Native Elements benefits from a vibrant and active community of developers. This means that there are regular updates, bug fixes, and a wealth of resources available for troubleshooting and learning.
- Scalability: As your mobile app evolves and grows in complexity, React Native Elements can accommodate your needs. It provides a wide range of components to handle various functionalities, from simple buttons to complex navigation menus.
Advantages of Using React Native Elements:
- Faster Development: By offering pre-built UI components and icons, React Native Elements significantly speeds up the development process. Developers can focus more on app logic and functionality rather than spending excessive time on UI design.
- Cost-Efficiency: The ability to target both iOS and Android platforms with a single codebase further saves resources.
- Improved User Experience: The use of consistent and visually appealing UI elements, along with customizable icons, enhances the overall user experience, making your app more engaging and user-friendly.
- Easier Maintenance: With a unified set of components, maintaining and updating your app becomes more straightforward. Changes to the UI can be made efficiently, and updates can be applied consistently.
- Wide Adoption: React Native Elements has gained widespread adoption in the React Native development community, making it a trusted choice for building mobile apps.
Integrating React Native Elements Icons
Icons play an important role in enhancing the visual appeal and user experience of mobile applications. React Native Elements icons seamlessly incorporate icons into its component library, providing an extensive collection for various purposes.
1. Accessing the Icon Library
- React Native Elements offers a diverse set of icons, ranging from basic to intricate social media logos.
- These icons are easily accessible through the designated components, allowing for effortless integration.
2. Customization and Scalability
- Icons can be customized to match the overall theme of the application, ensuring a harmonious visual experience.
- Scalability options ensure that icons retain their crispness and clarity across different screen sizes.
3. Interactive Functionality
- React Native Elements allow for seamless interaction with icons, enabling developers to incorporate intuitive gestures and actions.
Conclusion
React Native Elements revolutionizes the mobile app development landscape by providing a comprehensive toolkit for building cross-platform applications. With its intuitive components and seamless integration of icons, developers can create visually stunning and highly functional apps with ease. Embrace the power of React Native Elements and unlock a world of possibilities in your mobile development endeavors.
Frequently Asked Questions
To integrate React-Native Elements into your project, simply use the npm package manager and run the command npm install react-native-elements.
Absolutely! It's advisable to refer to the official documentation and explore the extensive community resources available for valuable insights and best practices.
Certainly! React Native Elements provides a plethora of styling options and supports theming to ensure that components align with your desired design language.
Indeed, React Native Elements is well-suited for projects of varying scales. Its component-based architecture and robust theming capabilities make it a versatile choice.
You can actively participate in the open-source community by reporting issues, submitting pull requests, and engaging in discussions on the official GitHub repository.
Yes, the React Native Elements community is highly active and responsive. You can seek assistance on platforms like Stack Overflow, Reddit, or the official Discord server.
Yes, React-Native Paper is versatile and can be used to develop various types of applications, including but not limited to social media platforms, e-commerce websites, and productivity tools.
Absolutely. While React Native Paper provides an extensive set of icons, you can also seamlessly incorporate your own custom icons.
You can contribute to the React-Native-Paper project by reporting issues, suggesting improvements, or even submitting pull requests on the official GitHub repository.